
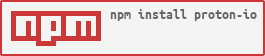
Getting Started
proton-io is a powerful bot framework for discord.js(v13). The package is designed to make developers work freely and easily.
if you are new proton-io this section for you!
First, you must be install proton-io using this node command:
npm install proton-io
if you are saying how can i use this module? don't panic. we write here some example:
const { ProtonClient } = require("proton-io");
const { Intents } = require("discord.js");
class Client extends ProtonClient {
super({
owners:["Client owner id(s)"],
intents: Object.values(Intents.FLAGS).reduce((p, c) => p + c, 0))
});
}
const client = new Client();
client.login("YOUR TOKEN HERE");
if you are look at some more guide codes visit here!
MongooseProvider
You can easily use your mongoose database with proton-io using Mongoose Provider.
Constructor
new Proton.MongooseProvider(model)
PARAMETER | TYPE | OPTIONAL | DEFAULT | DESCRIPTION |
---|---|---|---|---|
model | Mongoose Model | none | Connection for database. |
PROPERTIES
.model
mongoose model
Type: Model
METHODS
.set(datas)
data to be saved
Type: Object
//Example!
.set({
username:"Aktila",
id:"579592668208693260",
level:30
})
.get(data, ?defaultValue)
data to be get.
//Example!
.get({id:"579592668208693260"}, "test")
//return with
// datas: {
// username:"Aktila",
// id:"579592668208693260",
// level:30
//}
// if not exist return with "test";
.update(objectFetchDatas, updatedDatas)
a parameter to find data ordered by map, a parameter to update data ordered by map.
//Example!
.update({id:"579592668208693260"}, {level: 54})
//new level is 54!;
.delete(objectFetchDatas)
a parameter to find data ordered by map.
Type(s): Object
//Example!
.delete({id:"579592668208693260"})
//deleted.
.getall()
Fetch all model datas.
//Example!
.getall()
//All models data return with array.
MySqlProvider
You can easily use your mysql2 database with proton-io using MySql Provider.
Constructor
new Proton.MySqlProvider(connection)
PARAMETER | TYPE | OPTIONAL | DEFAULT | DESCRIPTION |
---|---|---|---|---|
connection | any | none | Connection for database. |
PROPERTIES
.connection
mysql2 connection
Type: mysql2 connection
METHODS
.set(id, savedDatas)
id to be saved
//Example!
.set("579592668208693260", {
level:23,
isActive:true
})
.get(id, ?defaultValue)
id to be get.
//Example!
.get("579592668208693260","test");
// return with datas:
// datas: {
// level:23,
// isActive:true
//} if not exist return with "test";
.update(id, updatedDatas)
a parameter to find data ordered by string, a parameter to update data ordered by map.
//Example!
.update("579592668208693260",{level: 50});
// new level is 50!
.delete(id)
a parameter to find data ordered by string.
Type(s): String
//Example!
.delete("579592668208693260");
// deleted.
SqlProvider
You can easily use your better-sqlite3 database with proton-io using SqlProvider.
Constructor
new Proton.SqlProvider(sql)
PARAMETER | TYPE | OPTIONAL | DEFAULT | DESCRIPTION |
---|---|---|---|---|
sql | any | none | Connection for database. |
PROPERTIES
.sql
sql filePath.
Type: sql filePath.
METHODS
.set(id, savedDatas)
id to be saved
//Example!
.set("579592668208693260", {
level:23,
isActive:true
})
.get(id, ?defaultValue)
id to be get.
//Example!
.get("579592668208693260","test");
// return with datas:
// datas: {
// level:23,
// isActive:true
//} if not exist return with "test";
.update(id, updatedDatas)
a parameter to find data ordered by string, a parameter to update data ordered by map.
//Example!
.update("579592668208693260",{level: 50});
// new level is 50!
.delete(id)
a parameter to find data ordered by string.
Type(s): String
//Example!
.delete("579592668208693260");
// deleted.
ProtonClient extends Client
ProtonClient
Constructor
new Proton.ProtonClient(options)
PARAMETER | TYPE | OPTIONAL | DEFAULT | DESCRIPTION |
---|---|---|---|---|
Options | ProtonClientOptions | none | Options for the client. |
ProtonHandler extends EventEmitter
ProtonHandler
Constructor
new Proton.ProtonHandler(client, options)
PARAMETER | TYPE | OPTIONAL | DESCRIPTION |
---|---|---|---|
client | ProtonClient | client for handler. | |
options | ProtonHandlerOptions | options for handler. |
PROPERTIES
.client
ProtonClient
Type(s): none
.options
Some Options for handler
Type: Object
METHODS
.register(mod,filepath)
It register the module in the cache.
Type(s): any, String
//Example!
.register(ProtonModule ,"./yourFilePath")
.deregister(mod,filepath)
It deregister the module from the cache.
Type(s): any, String
//Example!
.deregister(ProtonModule)
.load(fileOrFn)
Loads the module.
Type(s): String
or class
//Example!
.load("filePathOrClass")
.loadAll()
Loads the module.
Type(s):
//Example!
.loadAll()
.load(fileOrFn)
Loads the module.
Type(s): String
or class
//Example!
.load("filePathOrClass")
.reload(id)
Reloads the module.
Type(s): String
//Example!
//command id
.reload(id)
.reloadAll()
Reloads the module.
Type:
//Example!
.reloadAll()
.remove(id)
Remove the module.
Type: String
//Example!
.remove(id)
.removeAll()
Remove the module.
Type:
//Example!
.removeAll()
ProtonModule
ProtonModule
Constructor
new Proton.ProtonModule(id, options)
PARAMETER | TYPE | OPTIONAL | DESCRIPTION |
---|---|---|---|
id | ProtonModule | s. | |
options | ProtonModuleOptions | options for handler. |
PROPERTIES
.id
Command id
Type(s): none
.options
Options
Type(s): Object
METHODS
.execute() ABSTRACT
Executes the command.
Type(s):
.reload()
Reload the command.
Type(s):
.remove()
Remove the command.
Type(s):
ProtonClientOptions extends DiscordClientOptions
Types
ProtonHandlerOptions
Types
ProtonModuleOptions
Types
PARAMETER | TYPE | OPTIONAL | DEFAULT | DESCRIPTION |
---|---|---|---|---|
category | String | "default" | Category of the module. |